Using in combination with express
i18next will provide a middleware for express to use i18n inside your routes. In addition you could set 18next to add an app helper to use translations inside the serverside templates.
Express middleware
Register middleware:
var i18n = require("i18next");
i18n.init();
// Configuration block of express app
app.configure(function() {
app.use(express.bodyParser());
app.use(i18n.handle);
app.use(app.router);
// ...
});
Hint: To avoid some routes from beeing handled (detect lng, set cookie,...) you can add an array of strings that should be ignored.
i18n.init({
ignoreRoutes: ['images/', 'public/', 'css/', 'js/']
});
Access i18n inside your route:
app.get("/", function(req, res) {
// current language
var currentLng = req.locale;
// access i18n
var i18n = req.i18n;
// translate
var translation = i18n.t("my.key");
res.send(translation);
});
SEO friendly routes:
Ever wanted localized routes like:
- en: /en/products/harddrives/overview
- de: /de/produkte/festplatten/uebersicht
Just add routes like: i18n.addRoute(route, languages, expressApp, verb, routeFunction)
eg:
i18n.init({ preload: ['de', 'en']}, function(t) {
// after initialisation with preloading needed languages
// you could add localizable routes:
i18n.addRoute('/:lng/route.products/route.harddrives/route.overview', ['de', 'en'], app, 'get', function(req, res) {
// res.send(...);
});
});
route parts starting with ':' will be added as params. Every other part will be looked up as key, eg.:
- key 'route.products'
- key 'route.harddrives'
You can use this in combination with detecting the language from path:
i18n.init({ detectLngFromPath: 0 }); // default false
This will get language from first part of the route, in the example /:lng/
If no valid language is found i18next will try to get language via other options. You can avoid further language detection by setting 'forceDetectLngFromPath' to true on init options.
To only accept setting languages to values you have translated you could add option 'supportedLngs: ['en', 'de', 'fr']'.
Usage inside serverside Template
Register app.locals (app.helper):
var i18n = require("i18next");
i18n.init();
i18n.registerAppHelper(app);
// translate inside your template using t function (sample in jade)
body
span= t("my.key")
// get language sample in jade
body
span= i18n.lng()
Serve i18next for clientside usage
Serve clientscript and register routes:
i18n.serveClientScript(app) // grab i18next.js in browser
.serveDynamicResources(app) // route which returns all resources in on response
.serveMissingKeyRoute(app) // route to send missing keys
.serveChangeKeyRoute(app) // route to post value changes
.serveRemoveKeyRoute(app); // route to remove key/value
Authentication:
The 'postMissing', 'change' and 'remove' routes can be called with a second argument providing a function for authentifation, eg:
i18n.serveChangeKeyRoute(app, function(req, res) {
return !req.user;
});
Now you can use i18next on the client like:
// add script
script(src="i18next/i18next.js", type="text/javascript")
// init i18next in script block
i18n.init([options], function() {
$('#appname').text(i18n.t("app.name"));
});
// to support the dynamic route in client add options
i18n.init({
resGetPath: "locales/resources.json?lng=__lng__&ns=__ns__",
dynamicLoad: true
});
// to support posting of missing keys add option
i18n.init({
sendMissing: true
});
For details read the client api documetation.
Serve i18next-webtranslate
Serve i18next-webtranslate:
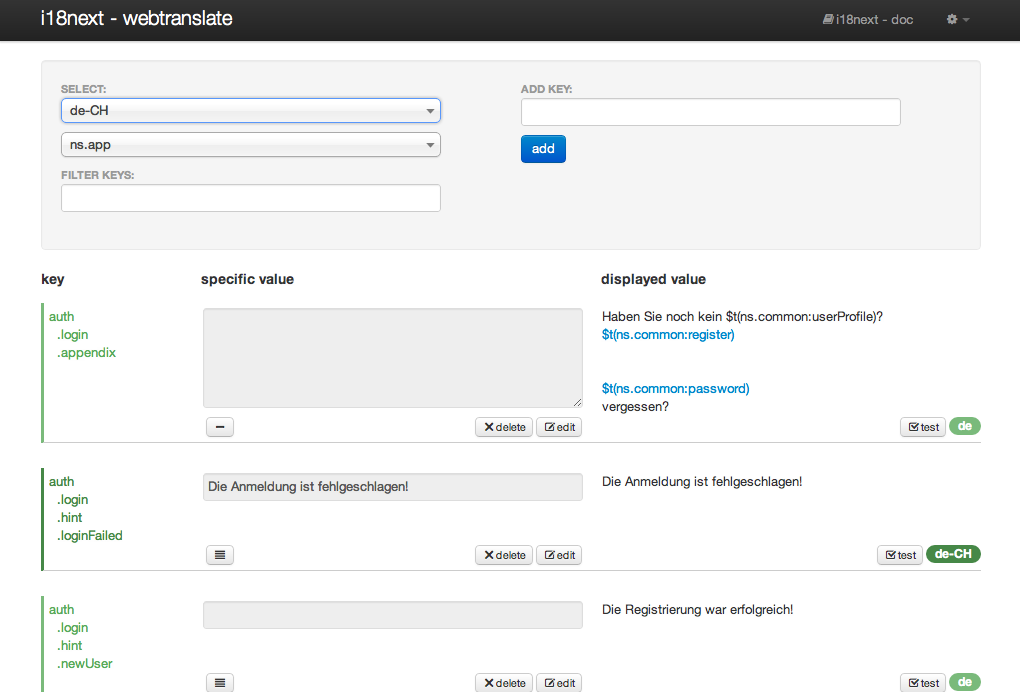
// i18next-webtranslate depends on following routes
i18n.serveChangeKeyRoute(app)
.serveRemoveKeyRoute(app);
// serve i18next-webtranslate
i18next.serveWebTranslate(app, {
i18nextWTOptions: {
languages: ['en_US', 'de', 'fr', 'it'],
namespaces: ['ns.app', 'ns.common', 'ns.layout', 'ns.msg', 'ns.public'],
resGetPath: "locales/resources.json?lng=__lng__&ns=__ns__",
resChangePath: 'locales/change/__lng__/__ns__',
resRemovePath: 'locales/remove/__lng__/__ns__',
fallbackLng: "en",
dynamicLoad: true
}
});
Authentication:
i18n.serveChangeKeyRoute(app,
i18nextWTOptions: {
languages: ['en_US', 'de', 'fr', 'it'],
// ....
},
authenticated: function(req, res) {
return !req.user;
}
);
Additional languages:
i18next-webtranslate comes with english and german translations.
i18n.serveChangeKeyRoute(app,
i18nextWTOptions: {
languages: ['en_US', 'de', 'fr', 'it'],
// ....
},
resourceSets: [{
language: 'en',
resources: {
layout: {
header: {
language: 'english' // will be displayed in language switch
}
},
editor: {
// ...
}
}
}]
);
For updated list of needed resource keys have a look here.